How to Uncomment Code in C: A Comprehensive Guide
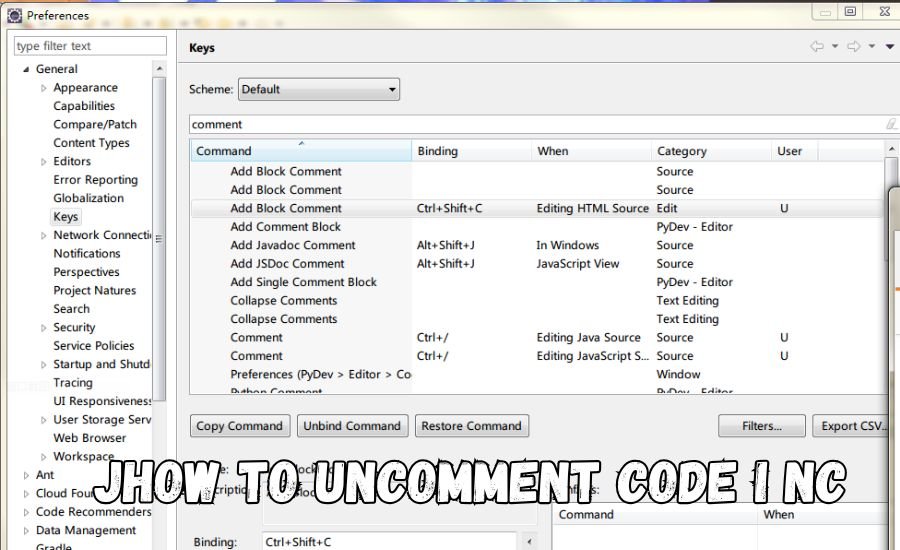
Programming languages have their own sets of rules and conventions. In C, comments are crucial for documentation and improving code readability. However, sometimes you need to uncomment code, meaning you remove comment markers to make the code executable again. This guide will explain how to uncomment code in C, with simple examples and practical tips.
What Are Comments in C?
Before we dive into uncommenting, let’s understand what comments are in C. Comments are pieces of code that the compiler ignores. They help you explain your code without affecting its execution. In C, there are two types of comments:
Single-line comments: These comments start with //. Everything after // on that line is ignored by the compiler.
c
Copy code
// This is a single-line comment
printf(“Hello, World!”); // This prints Hello, World!
Multi-line comments: These comments start with /* and end with */. You can use them for longer explanations or to comment out multiple lines.
c
Copy code
/* This is a multi-line comment.
It can span multiple lines. */
printf(“Hello, World!”);
Why Would You Want to Uncomment Code?
Uncommenting code can be necessary for several reasons:
- Testing: You may want to temporarily disable certain parts of your code for testing purposes.
- Debugging: Uncommenting code can help you identify bugs or issues in your program.
- Refactoring: When you improve or change your code, you might need to uncomment sections to integrate them into the new structure.
How to Uncomment Code in C
Now that we understand the role of comments in C, let’s look at how to uncomment code. The process is straightforward and depends on the type of comment.
Uncommenting Single-Line Comments
To uncomment a line that is commented out with //, simply remove the // at the beginning of the line.
Example:
c
Copy code
// printf(“This line is commented out.”);
printf(“This line is active now.”);
Uncommented:
c
Copy code
printf(“This line is active now.”);
In the above example, removing // from the first line activates it.
Check Out the Latest Blogs Regarding: Ramit-Kalia-Patent
Uncommenting Multi-Line Comments
For multi-line comments, you need to remove the /* at the beginning and the */ at the end of the comment block.
Example:
c
Copy code
/*
printf(“This line is commented out.”);
printf(“This line is also commented out.”);
*/
printf(“This line is active now.”);
Uncommented:
c
printf(“This line is commented out.”);
printf(“This line is also commented out.”);
printf(“This line is active now.”);
Here, both lines that were commented out are now active after removing the comment markers.
Best Practices for Commenting and Uncommenting Code
While commenting and uncommenting code is essential, it’s crucial to do it correctly to maintain code quality. Here are some best practices:
- Be Clear and Concise: Make sure your comments explain what the code does without being too long. Use simple language that anyone can understand.
- Avoid Obvious Comments: Don’t comment on what is already clear from the code. For example, don’t write // Increment i next to i++;.
- Use Comments for Complex Logic: If you have complex code that may confuse others, add comments explaining the logic.
- Regularly Review and Update Comments: As you change your code, make sure your comments are still accurate. Outdated comments can mislead others (or yourself).
- Uncommenting Carefully: When uncommenting, be sure the code is still functional. Test the uncommented code to ensure it works as expected.
Common Mistakes When Commenting and Uncommenting
- Not Testing After Uncommenting: Always test your code after uncommenting to ensure it still functions correctly.
- Removing Comments That Provide Value: Sometimes, comments explain the rationale behind a decision. Removing them can cause confusion later.
- Overusing Comments: Too many comments can clutter your code. Aim for a balance where comments enhance understanding without overwhelming the reader.
Conclusion
Uncommenting code in C is a simple yet essential skill for programmers. By understanding the different types of comments and how to remove them properly, you can maintain a clean and effective codebase. Remember to follow best practices when commenting and uncommenting, and always test your code after making changes.
This guide provided you with a comprehensive look at how to uncomment code in C. By following these steps and tips, you’ll ensure that your code remains clear, maintainable, and effective.
FAQs
Q: What is the difference between single-line and multi-line comments?
A: Single-line comments begin with // and are used for brief explanations, while multi-line comments start with /* and end with */, allowing for longer text and multiple lines.
Q: Why should I comment my code?
A: Comments help explain your code, making it easier for others (or yourself) to understand its purpose and logic. They are especially useful for complex sections of code.
Q: Can I uncomment a section of code that contains syntax errors?
A: Yes, you can uncomment code with syntax errors, but the program will not compile or run correctly until those errors are fixed.
Q: Is there a limit to how many comments I can add?
A: There’s no official limit, but too many comments can clutter your code. Aim for clarity and conciseness.
Read More: Kyomitorret-Blogspot-Com